Yunus Emre
Software Engineer
Flutter Sharepoint Data Pull - RestApi Bearer
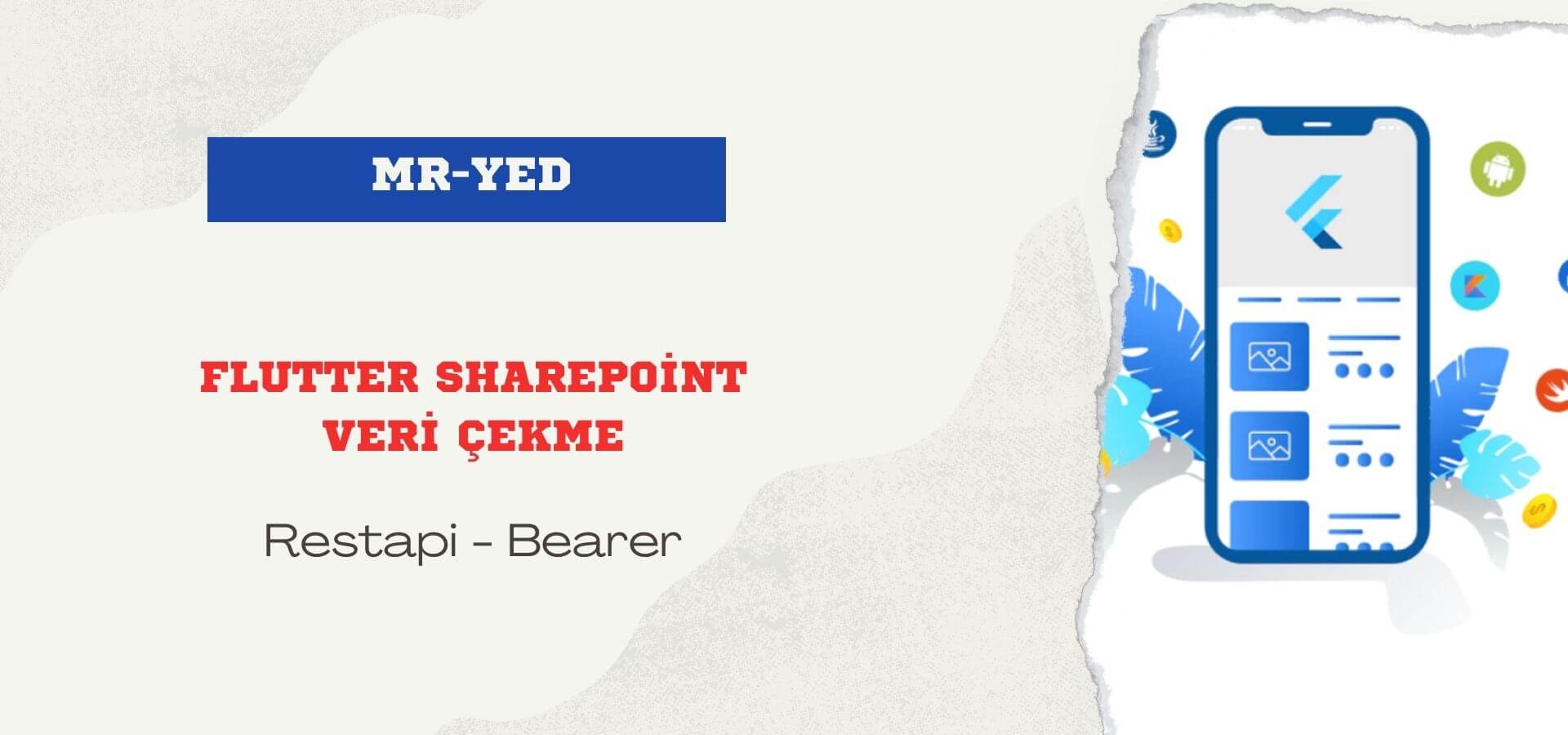
Recently, I needed to pull data from Sharepoint lists with C# for a few projects. Then I wanted to do the same with Flutter. For this reason, I will explain the Flutter sharepoint data extraction process in this article.
Flutter Sharepoint Data Pull
With Flutter, we can pull the data we want from our sharepoint lists. To do this, we first create application information with the link below. "Mryed" is my site name in the sharepoint application. You can write the name of your own site there.
https://{tenantname}.sharepoint.com/sites/Mryed/_layouts/15/appregnew.aspx
You can write down the client ID and secret key from the "appregnew" page in a text document. I wrote "localhost" in the application domain as a test and "https://localhost" in the URL field. Afterwards;
https://{tenantname}.sharepoint.com/sites/Mryed/_layouts/15/appinv.aspx
page and write the application ID we noted on the previous page here. When you press the search key, the fields under that application ID will be filled. We write the following code in the "Permission Request XML:" field at the bottom of the form.
<AppPermissionRequests AllowAppOnlyPolicy="true">
<AppPermissionRequest Scope="http://sharepoint/content/sitecollection"
Right="FullControl" />
</AppPermissionRequests>
More details for Sharepoint permission XML codes; Medium.
After clicking the Create button, there will be a confirmation field that says "Do you trust the {Title} application?". Click on the "trust" button and we will be redirected to the "Site Settings" page. On the site settings page, we enter the "Site collection application permissions" field. You can view the application permission you created here. You can take note of the field written in the application identifier column here. We will especially use the part after the "@" sign.
After this stage, we are done with the sharepoint side. What we need now is the Bearer token we need to connect from Flutter. I use the postman application for this. We enter the link below in the postman url field. The part that says code is the area after the "@" sign that we note.
https://accounts.accesscontrol.windows.net/{kod}/tokens/oAuth/2
Then we come to the body field in postman. The client ID and secret key are the information we jot down in the text document in the first step. The code is again the part after the "@" sign.
grant_type:client_credentials
client_id:{istemci kimliği}
client_secret:{gizli anahtar}
resource:00000003-0000-0ff1-ce00-000000000000/{tenant}.sharepoint.com@{kod}
Now we can click on the "Send" button. Here we will get a list with "token_type": "Bearer" in the list. Here we copy the long code in the "access-token" field into the text document.
Flutter RestApi
Now that we have the access token information, we can switch to the flutter side. Paste the code "access-token" into the string value named tokenValue. There will be a space after Bearer and after the space will be your access token information. Don't forget to write your own tenant information in the sharepoint link in Uri.parse.
In the code below, I pulled the "name, surname, registration" information from the "Personnel" list on the "Mryed" site. You can change the code according to the data you want to pull.
import 'dart:convert';
import 'package:http/http.dart' as http;
class Personel {
final String adSoyad;
final String sicil;
Personel({required this.adSoyad, required this.sicil});
factory Personel.fromJson(Map<String, dynamic> json) {
return Personel(
adSoyad: json['AdSoyad'],
sicil: json['Sicil'],
);
}
static Future<List<Personel>> getPersonelList() async {
String tokenValue =
"Bearer {token alanı}";
final response = await http.get(
Uri.parse(
'https://{tenant}.sharepoint.com/sites/MrYed/_api/web/lists/getbytitle(\'Personel\')/items?\$select=AdSoyad,Sicil'),
headers: {
'Accept': 'application/json;odata=verbose',
'Authorization': '$tokenValue'
});
if (response.statusCode == 200) {
print("Basarili");
final data = jsonDecode(response.body)['d']['results'] as List<dynamic>;
return data.map((item) => Personel.fromJson(item)).toList();
} else {
throw Exception('Failed to load data');
}
}
}
That's it for Flutter Sharepoint data extraction. In the following stages, I can do sharepoint data extraction, deletion and update operations with flutter. See you in the next article content...
2 Comments